Ansible modules are the foundation of Ansible’s automation capabilities. They are small programs that perform specific tasks, enabling you to automate everything from software installation to cloud provisioning. This article explains what Ansible modules are, their types, and how to use them in playbooks.
What Are Ansible Modules?
Ansible modules are standalone units of code executed by Ansible to perform specific tasks on managed nodes. These tasks can range from system configuration and file manipulation to cloud infrastructure provisioning.
Modules are also referred to as “task plugins” or “library plugins” and are invoked in Ansible playbooks as tasks.
Key Features of Ansible Modules:
- Idempotence: Modules are designed to achieve the same result regardless of how many times they are run.
- Agentless Execution: Modules execute over SSH or WinRM without requiring agents on the target systems.
- Extensibility: You can create custom modules to extend functionality.
Types of Ansible Modules
Ansible includes a wide variety of modules categorized by their functionality:
1. Core Modules
- Maintained by the Ansible team and included in all installations.
- Examples:
file
,user
,service
,package
.
2. Cloud Modules
- Manage resources in cloud platforms like AWS, Azure, and Google Cloud.
- Examples:
amazon.aws.ec2
,azure.azcollection.azure_rm_vm
.
3. Networking Modules
- Automate network device configurations.
- Examples:
ios_config
,junos_config
,nxos_command
.
4. Database Modules
- Manage databases like MySQL, PostgreSQL, and MongoDB.
- Examples:
mysql_user
,postgresql_db
.
5. Custom Modules
- User-created modules to meet specific requirements.
Anatomy of an Ansible Module Task
Here’s an example task using the apt
module to install Nginx:
- name: Install Nginx
apt:
name: nginx
state: present
Task Components:
name
: A description of the task.apt
: The module used for the task.name
: The package to be installed.state
: Ensures the package is installed.
Commonly Used Ansible Modules
File Management
copy
: Copy files to target machines.template
: Deploy Jinja2 templates.file
: Manage file and directory properties.
Service Management
service
: Start, stop, and manage services.systemd
: Interact with systemd services.
Package Management
yum
: Manage packages on RHEL-based systems.apt
: Manage packages on Debian-based systems.
Cloud and Virtualization
ec2
: Provision AWS EC2 instances.vmware_guest
: Manage VMware virtual machines.
User Management
user
: Create, update, or delete user accounts.group
: Manage user groups.
Writing Custom Ansible Modules
Custom modules can be written in Python, PowerShell, or any scripting language. A simple Python module follows this structure:
#!/usr/bin/python
from ansible.module_utils.basic import AnsibleModule
def main():
module = AnsibleModule(
argument_spec=dict(
name=dict(type='str', required=True)
)
)
response = {"message": f"Hello {module.params['name']}!"}
module.exit_json(changed=False, **response)
if __name__ == '__main__':
main()
Using Modules in Playbooks
Modules are called in tasks within a playbook. Example playbook to ensure a service is running:
- hosts: webservers
tasks:
- name: Ensure Nginx is running
service:
name: nginx
state: started
Conclusion
Ansible modules are the essential building blocks of Ansible automation. Whether you’re managing servers, deploying applications, or provisioning cloud resources, modules enable you to perform tasks efficiently and consistently.
Explore More About Ansible Modules in the Official Documentation
Subscribe to the YouTube channel, Medium, and Website, X (formerly Twitter) to not miss the next episode of the Ansible Pilot.Academy
Learn more with in-depth examples in Ansible by Examples.
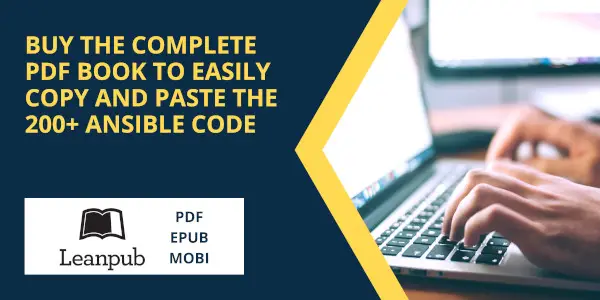
Donate
Support the author and this project by donating.